You will never see your code like before once you understand JIT. In this article we will demonstrate how JIT generates IL code, by comparing performance of List and generics by demonstrating how JIT generated IL code is created and its use.
What happens when you run the test application for performance comparison?
- When you run the application, JIT (Just in Time) compiler runs.
- JIT compiles the application, optimizes the code, and finds the best approach to executes the code.
- All these JIT steps are time-consuming which are included in the first execution, so the first execution time is always higher than the actual run time
- So how can you calculate the actual run time? Simple! To check the performance of your test application with test data, call the code snippet in a loop.
Example code
private static void GenericListPerformance() {
var genList = new List < int > () {
10,
100,
13,
19,
14,
100,
99
};
var nonGenList = new ArrayList() {
10,
100,
13,
19,
14,
100,
99
};
var stWatch = Stopwatch.StartNew();
genList.Sort();
stWatch.Stop();
Console.WriteLine("Generic List sorting " + stWatch.Elapsed.TotalMilliseconds.ToString());
stWatch = Stopwatch.StartNew();
nonGenList.Sort();
stWatch.Stop();
Console.WriteLine("Non Generic List sorting " + stWatch.Elapsed.TotalMilliseconds.ToString());
}
Here, I have created a simple method to compare the two lists – ArrayList and List<T>. I have used Stopwatch which is present in the System.Diagnostics namespace of .NET framework and then, printed the messages with time.
static void Main(string[] args) {
for (int i = 0; i < 5; i++) {
Console.WriteLine(string.Format("<--------Call Number {0} -------->", i));
GenericListPerformance();
Console.WriteLine();
}
}
Now, here I have used the actual concept. I called the same method in main, inside a loop. So, when it runs for the first time, JIT can optimize the code and find the best approach to run this code. Later, JIT will use the native code and give us the best results.
Result
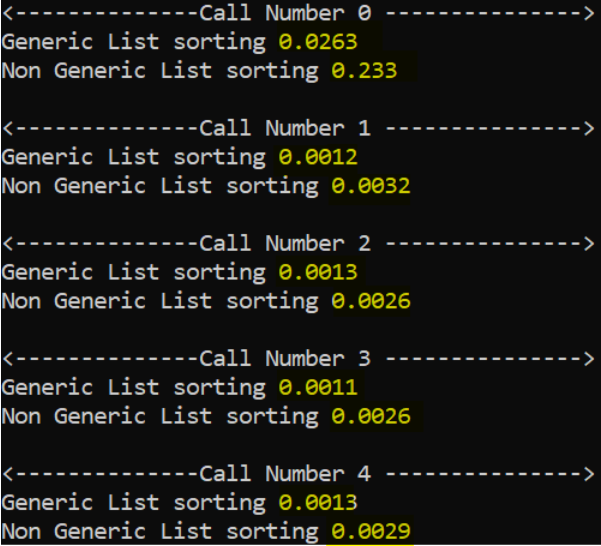
performance of List
The first run, the execution time is-
For Generic – 0.0263
For Non-Generic – 0.233
And later, it came to be like –
Generic – 0.0012, 0.0013, 0.0011, 0.0013
Non-Generic – 0.0032, 0.0026, 0.0026, 0.0029
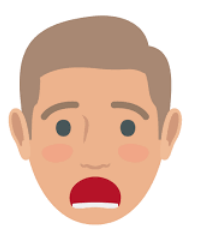
Therefore, we should always consider the JIT time.
That is it. Comment if you have any questions or want me to do some analysis on a topic you want.
More like this
- How does a dictionary maintains data
- What is better between Enumerator or foreach
- How to access private member of class
- Better make use of Service bus Queue and Topic
- How to trigger azure service bus trigger functions from postman
Natalia Morris
Sonny Pope
Gracie Chang
Milani Hoffman
Maya Cervantes
Phoenix Rhodes
moncia stratmann
Cartier Brock
arseno wintterlin
doralia winninger